Interacting
Exchanges should integrate the IBC token (ICS-20) of the RollApp on Dymension. This approach is identical to an IBC token (ICS-20) on Cosmos Hub or Arbitrum tokens (ERC-20) on Ethereum L1.
info
Make sure you have successfully integrated the Dymension blockchain according to best practices here.
RollApp token address
Interacting with tokens on Dymension can be done with 2 “parallel” standards: IBC (ICS-20) and ERC-20, BOTH SHARE THE SAME STATE, regardless of the method chosen.
IBC standard
Example: USDC on Dymension ibc/B3504E092456BA618CC28AC671A71FB08C6CA0FD0BE7C8A5B5A3E2DD933CC9E4
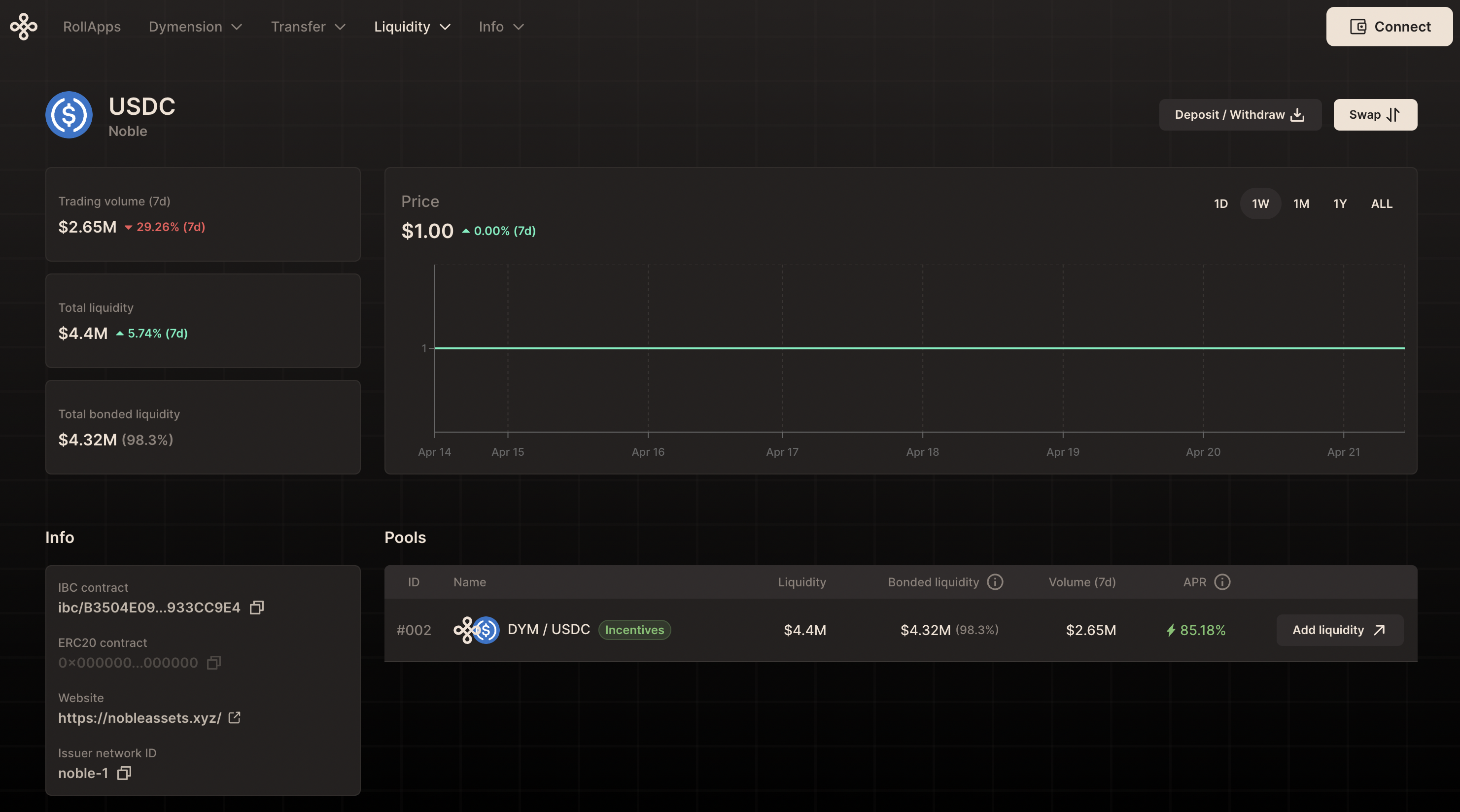
Singing and Broadcasting using cosmJS:
import { DirectSecp256k1HdWallet } from "@cosmjs/proto-signing";
import { GasPrice, SigningStargateClient } from "@cosmjs/stargate";
import { Decimal } from "@cosmjs/math";
const signer = await DirectSecp256k1HdWallet.fromMnemonic("<mnemonic>", {
prefix: "dym",
});
const gasPrice = new GasPrice(Decimal.fromUserInput("20000000000", 18), "adym");
const client = await SigningStargateClient.connectWithSigner("<rpc>", signer, {
gasPrice,
});
const fromAddress = (await signer.getAccounts())[0].address;
const coins = [{ denom: "<denom-ibc-hash>", amount: "<amount>" }];
const response = await client.sendTokens(
fromAddress,
"<to-address>",
coins,
"auto"
);
ERC-20 contract standard
ERC-20 Standard on Dymension is only a virtual adapter of the Cosmos bank modules.
Singing and Broadcasting using ethers:
import { ethers } from "ethers";
const signer = ethers.Wallet.fromMnemonic("<mnemonic>");
// or another option:
// const signer = (new ethers.providers.JsonRpcProvider('<json-rpc>')).getSigner('<signer-address>');
const transferABI = [
{
inputs: [
{
internalType: "address",
name: "recipient",
type: "address",
},
{
internalType: "uint256",
name: "amount",
type: "uint256",
},
],
name: "transfer",
outputs: [
{
internalType: "bool",
name: "",
type: "bool",
},
],
stateMutability: "nonpayable",
type: "function",
},
];
const contract = new ethers.Contract(
"<erc20-contract-address>",
transferABI,
signer
);
const result = await contract.transfer("<to-address>", "<amount>");